Gatsby Page Query Variables
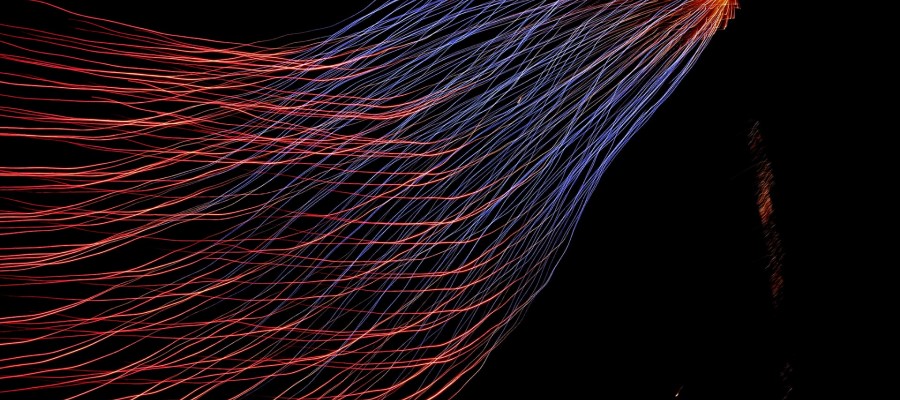
I felt the need to add paging to the blog list. As I started down this path, I knew that during the implementation of this feature there would be something that I did not yet know. In past Gatsby implementations and components, I have used a graphql
data results to generate pages for the site. But, I had never had to pass any data into that query. Resulting in a different set of data points for each page. How about a problem statement to get started?
As a consumer of blog posts, I need a list of blog entries to choose from in a format that loads quickly and is easily understood.
To perform this, there was a semi-drastic refactor to the existing blog list page. Moving it from a concrete implementation in the pages
directory to a template. Where the template is used to generate several blog list pages in the final distributable directory. The graphql page query to return all blog entries started like this.
export const query = graphql`
query blogPageQuery {
allMdx(
sort: {order: DESC, fields: [frontmatter___date]}
) {
edges {
node {
... properties to populate the card ...
}
}
}
}
}
`;
To implement paging, additional variables have to be added. The number of posts to $skip
and a $limit
to the number of blog posts to take. The refactored with the variables looks something like this.
export const query = graphql`
query ($skip: Int!, $limit: Int!) {
allMdx(
sort: { order: DESC, fields: [frontmatter___date] }
limit: $limit
skip: $skip
) {
edges {
node {
... properties to populate the card ...
}
}
}
}
}
`;
Notice the definition of the variables in the query ($skip: Int!, $limit: Int!)
then the consumption of those variables in the allMdx
query arguments. Not so difficult to understand the query, but how do we populate those variables as the pages are built?
In the gatsby-node.js
is where we can generate the pages in the site. As part of the object passed to the createPage
function, we can also pass context
to the page being created. By default, Gatsby will take the data passed into the context
object and attempt to find properties named the same as they are in the graphql query.
for (var i = 0; i < blogPageCount; i++) {
createPage({
path: getBlogListPagePath(i + 1),
component: blogListTemplate,
context: {
skip: i * blogListPageSize,
limit: blogListPageSize,
// Other context passed into the page
},
});
}
With the skip
and limit
properties on the context, all that is left is to provide each property with a valid integer value. Restarting the gatsby development server several pages blog list pages are built and ready for distribution.