Azure Functions - Cosmos Trigger
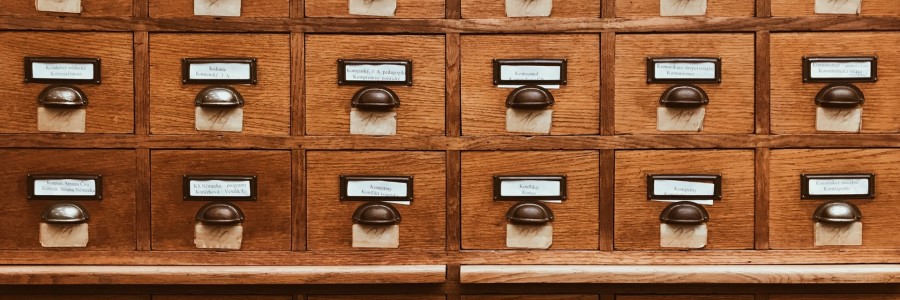
Raise your hand if you have ever implemented a trigger in some database technology ✋. What did you think of that experience? Did you hit a wall with the ability that database triggers offer? What if you wanted to signal another system that data was updated? To my knowledge, you can not do that with a database trigger.
If you are using Cosmos, this can be done with Azure Functions! You can perform nearly any action you so desire after an entity is created or updated in Cosmos, with this approach. Let's jump into the sample then compare it to other Azure Function triggers:
[FunctionName("CosmosAudit")]
public static void Run([CosmosDBTrigger(
databaseName: "FunctionContainer",
collectionName: "person",
ConnectionStringSetting = "CosmosConnectionString",
CreateLeaseCollectionIfNotExists = true)]IReadOnlyList<Document> peopleDocuments,
ILogger log)
{
if (peopleDocuments != null && peopleDocuments.Count > 0)
{
log.LogInformation($"Documents Audited : {peopleDocuments.Count}");
foreach (var personDocument in peopleDocuments)
{
log.LogInformation($"{personDocument.Id} : {personDocument.GetPropertyValue<string>("firstName")} : {personDocument.GetPropertyValue<string>("lastName")}");
}
}
}
As with all the other sample implementations in this series, the main difference in each is the first parameter of the method. For this trigger, the parameter is a read-only list of Cosmos Document
. This parameter is decorated with CosmosDBTrigger
. The annotation constructor here takes in quite a few arguments compared to the others that have been sampled in this series. Let's quick break down those arguments:
databaseName
: Just as in SQL Server, all data wrapped in 2 constructs. The upper most construct in Cosmos is referred to as a database or container. This is used to group like things together.collectionName
: Similar to SQL Server, there is a child within the outer-most container. In SQL Server, this construct is a table. In Cosmos, this construct is a container.ConnectionStringSetting
: The configuration key containing the information for the trigger to connect to the Cosmos DB.CreateLeaseCollectionIfNotExists
: A lease collection is the mechanism that maintains the state across several Azure Functions, the lease collection is required to establish a connection between Cosmos and the Azure Function trigger. A lease collection can be created manually, however, for this example the connection is what we are looking for. Not, understanding the manual creation of a lease collection.
As far as this trigger is concerned, the implementation is not all that interesting. For each of the document arguments the Id, first name and last name are logged. But, this could be anything! For instance, for each new person added to the collection, we could email them welcoming them to the platform. Or we could write all the data changes to a different audit application. Perhaps, we use this data to increment a counter to capture how many application writes happen throughout the entire system. The possibilities are endless!