Azure Functions - Web API JavaScript
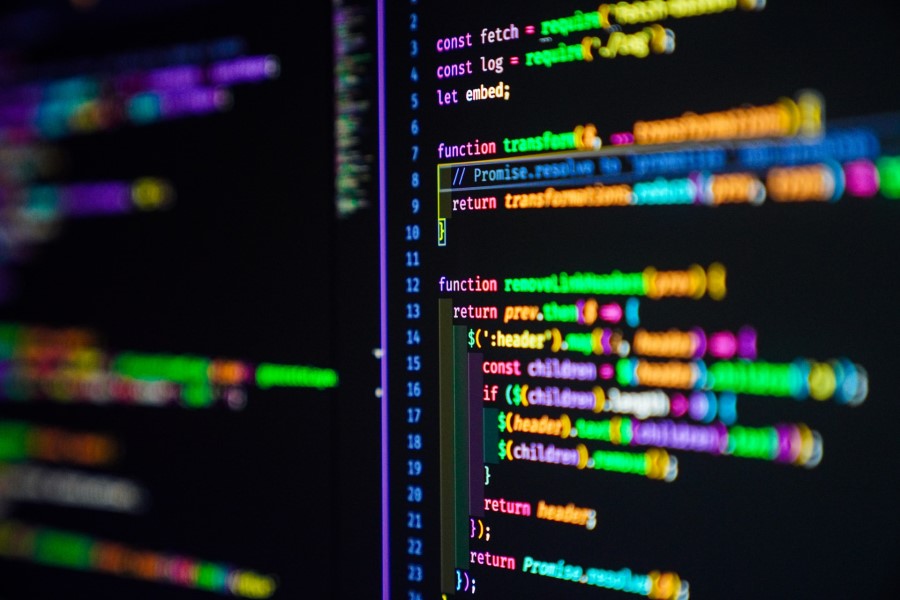
In taking a deeper dive into the world of Azure Functions, I noted that there is language support beyond .NET technologies. One of those supported languages, JavaScript! JavaScript does not particularly excite me, but I am interested in the ability to quickly prototype as it is normally
quick to get something up and working. Since this is all for learning, just how quickly can one create a JavaScript API using hosting it as an Azure Function?
First, a quick discussion about tooling. I have been using VS Code to do a lot in the past year or so. The extensions available for this tool are ever-improving. Two extensions, in particular, were of assistance. The first Azure Resource. This extension allows for the quick template of the project and function implementations. The other extension is Azure Functions Extension. The Azure Functions Extension allows the developer to easily execute the Azure Function implementations locally for testing and debugging.
Now with the additional tools to perform the implementation, I decided to implement the same web API as I did in Part 1 of this series. Let's take a look at the implementation, then compare the differences:
module.exports = async function (context, req) {
const responseMessage = { currentDateTime: new Date() };
context.res = {
body: responseMessage,
};
};
Seven lines of JavaScript is all it takes! This could even be cut down to five lines if we were looking to optimize file lengths. But, we are missing something here that the C# implementation has baked into it. Recall, the C# implementation is:
public static class DateTimeFunction
{
[FunctionName("datetime")]
public static IActionResult GetDateTime(
[HttpTrigger(AuthorizationLevel.Function, "get", "post", "put", "patch", "delete")] HttpRequest req,
ILogger log)
{
return new OkObjectResult(new { CurrentDateTime = DateTime.Now });
}
}
Making the comparison
The annotations in the C# implementation contain all the hooks to configure the Azure Function. When a JavaScript implementation is created using the extensions discussed above, there is an additional file created. A function.json
file, since JavaScript does not have an annotation mechanism out of the box, this configuration all lives in this additional file. Here is what the binding looks like:
{
"bindings": [
{
"authLevel": "anonymous",
"type": "httpTrigger",
"direction": "in",
"name": "req",
"methods": [
"get",
"post",
"put",
"patch",
"delete"
],
"route": "datetime"
},
{
"type": "http",
"direction": "out",
"name": "res"
}
]
}
The majority of what is defined in this file should look quite familiar, such as the type of httpTrigger
, the ability to set authLevel
for the endpoint, the list of methods
allowed, and the ability to name the route
to the endpoint. There are a couple of differences, notice the bindings
property is a list of objects. direction
is the first property in the objects that is not familiar. The acceptable values for this property are in
or out
defining the flow of the data from the Azure Function. Obviously, in
is the binding for data coming into the Azure Function. out
is the binding for the data being returned from the Azure Function. Then there is the name
property. name
defines the name of the variable to be used for the data source. Notice the second argument in the endpoint definition req
and how it matches the in
binding. Now notice the name res
on the second binding and the name of the property on the context
in the controller. Pretty slick binding.
Other differences
So the question now is, what are the other differences between a C# and JavaScript implementations. The main discovery is that only one Azure Function implementation can be per directory. By default the file structure is as follows:
- FunctionCollection (directory)
- FunctionImplementation (directory)
- function.json
- index.js
- host.json
- package.json
- FunctionImplementation (directory)
Where you can have n number of uniquely named FunctionImplementation
directories, each containing the implementation of a single Azure Function. Unlike the C# world where there can be many functions defined in one class file.
Another difference is the naming of the Azure Function. In C# you have to annotate the class method with the [FunctionName("datetime")]
attribute. Given that JavaScript does not have annotations comparable to C#, other files establish the Azure Function definition. Specifically, the host.json
file, which is a metadata file containing configuration options that are applied to all Azure Functions within the instance. With these configuration files, the default name for the Azure Function is the directory name, in the directory structure above would be FunctionImplementation
. From what I can find there is no opportunity to change this name with configuration.
Final thoughts
Given I come from a C# background, with very little NodeJS development in my experience. I was quite impressed how quickly the VS Code extensions got me from knowing very little to a basic understanding. Of course, the Microsoft Docs helped to fill in the gaps as well. I can see using this in the future to prove out a concept, or to shell out an API to play with. But I don't see me deploying a production-ready app using JavaScript as the service layer.