Azure Functions - Schedule Trigger
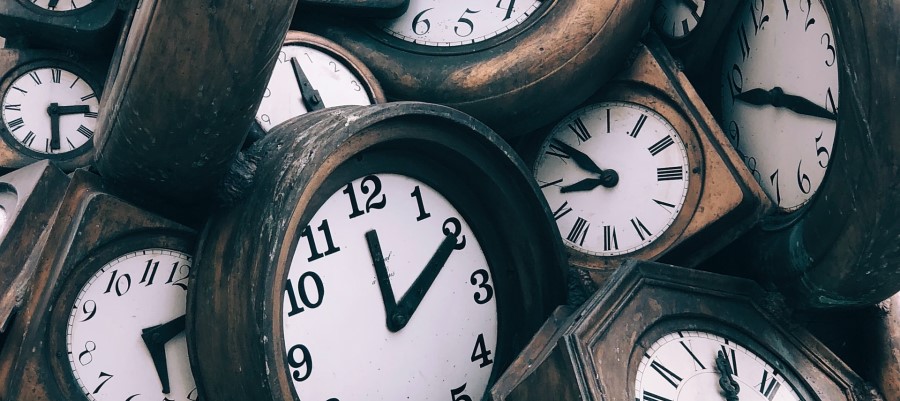
First a quick plug, this is just one post in a series of posts on Azure Functions. Check out the first post here. then, follow that one through all the content!
If you have any amount of software development experience, there is likely a time where there was a need to schedule an activity to occur. If not, I am shocked! As this is such a common need in the industry. Since we have already seen an example Azure Function implementation in my web API post let us just jump into the implementation.
[FunctionName("websiteping")]
public async Task Run(
[TimerTrigger("*/30 * * * * *")] TimerInfo myTimer,
ILogger log)
{
var client = HttpClientFactory.Create();
log.LogInformation("HttpClient Created");
var response = await client.GetAsync(new Uri("https://google.com"));
if (!response.IsSuccessStatusCode) log.LogCritical($"Website ping received unsuccessful response of: {response.StatusCode}");
log.LogInformation($"Website ping received successful response of: {response.StatusCode}");
}
At a high level, this is a quick monitor that makes an HTTP request to Google.com. If the response is not successful, log a critical message with the status code. Otherwise, log some information about the status code received.
Scanning through the implementation, the FunctionName
attribute on the method is the same as our web API implementation. The method name and return type are the same. The attribute decorating the first argument is where the magic happens. In the case of a timer event Azure Function, we use the TimerTrigger
attribute. But, what is that strange-looking string being passed into the constructor? That my friends is an NCronTab expression! A more detailed explanation of NCronTab can be found in the Azure Docs. Long story short, this expression is a space-delimited string with 6 fields. The fields represented are {second} {minute} {hour} {day} {month} {dayOfWeek}
. In the example snippet above */30 * * * * *
, the implementation will execute every 30th second of every minute, of every hour, of every day.
In using this event trigger, the implementation is quick, the scheduling is as fine-grained as you need or want. Making the possibilities endless for these types of implementation. So the next time you need to schedule some activity, why not take a look at deploying an Azure Function with a timer trigger?