Adding Content to My New Gatsby Site
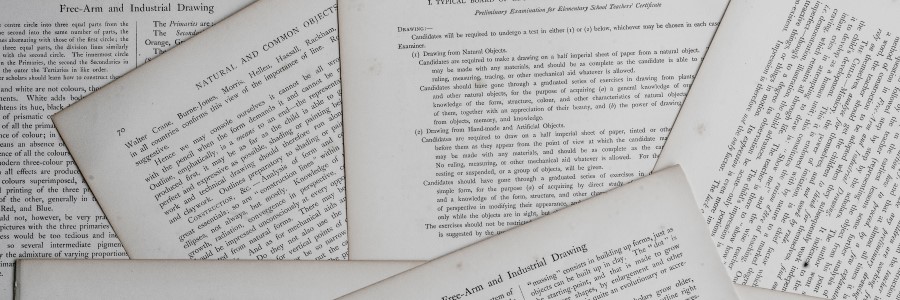
Before releasing this hot new content, if you have not read my previous posts on Gatsby. Please, get caught up with us so you too can follow along. The posts can be found here and here. With the shell of a site, how do we go about modifying the default page? This is quite easy if you are already familiar with React and JSX. If that is not the case, here is the react documentation and the documentation on JSX. In the remainder of this post, I'm continuing forward under the assumption that some of the above is understood.
First, let's start with modifying the default page that came out of the box. Locate /src/pages/index.js
. Recall in the last post in the series, where the /src/pages
directory is special in the Gatsby environment? For now, you should assume that every page will live in this directory. To start, open the command prompt to the current Gatsby working directory. Execute the command gatsby develop
, a handful of messages will be output in the command prompt window. Once the messages have appeared to stop writing, open a browser to localhost:8000
. The default page from the installer should resolve in the browser. Next, let's remove all the styles at the top of this file and most everything in the component. The file should look something like this when everything has been removed:
import * as React from "react"
const IndexPage = () => {
return (
<main></main>
)
}
export default IndexPage
Assuming the gatsby develop
command is still executing in the background. Once the file is saved, the CLI tooling will begin to build the minified and bundled files, then force a browser refresh. The new page should not have anything on it, but a blank white view, not very interesting. So let's add some of our content. Add a couple <div>
tags to our <main>
tag and some text. This is what I came up with:
import * as React from "react"
const IndexPage = () => {
return (
<main>
<div>Andrew's Site</div>
<div>Here is some super exciting content!</div>
</main>
)
}
export default IndexPage
As the file is saved, the presentation in the browser is updated as well. There you have it! Fresh new content in your Gatsby site. However, a single-page site is not all that interesting. How about we add another page, maybe two? Those are both really easy requests, in /src/pages/
add a file page1.js
with this as the content of the file:
import * as React from "react"
const PageOne = () => {
return (
<main>
<div>Andrew's Site</div>
<div>Page 1</div>
</main>
)
}
export default PageOne
As a sibling to the index.js
and page1.js
file, create a directory named new-directory
then add a file to the directory named page2.js
with this as the content of the file:
import * as React from "react"
const PageTwo = () => {
return (
<main>
<div>Andrew's Site</div>
<div>Page 2</div>
</main>
)
}
export default PageTwo
Now see how the CLI tooling responded, navigate to localhost:8000/page1
and localhost:8000/new-directory/page2
. Both pages should have been resolved without issue and you should see the content copied and pasted from above rendered in the browser. One thing you may have noticed is the text in the first <div>
is on all pages. Is there not a way that we can configure that text somewhere so we do not have to maintain the text on 3 different pages? The answer is OF COURSE! Open up the gatsby-config.js
file at the top of the file you should see some configuration for siteMetadata
perhaps, it looks something like this:
module.exports = {
siteMetadata: {
siteUrl: "https://www.yourdomain.tld",
title: "gatsby-blog-post",
},
};
Add a new line to the siteMetadata
object, something like this:
module.exports = {
siteMetadata: {
siteUrl: "https://www.yourdomain.tld",
title: "gatsby-blog-post",
pageHeading: "Andrew's Site",
}
};
After saving this change, you will have to stop the development server and restart it. So in command prompt, enter ctrl
+ c
then hit the up arrow and Enter
the server is now "bounced"
Update index.js
file contents to:
import * as React from "react";
import { graphql } from "gatsby";
const IndexPage = ({ data }) => {
return (
<main>
<div>{data.site.siteMetadata.pageHeading}</div>
<div>Here is some super exciting content!</div>
</main>
);
};
export const query = graphql`
query indexPageQuery {
site {
siteMetadata {
pageHeading
}
}
}
`;
export default IndexPage;
What is that on lines 13-21?
export const query = graphql`
query indexPageQuery {
site {
siteMetadata {
pageHeading
}
}
}
`;
That is GraphQL. This will be the topic of the next post on Gatsby. But for now, take a look at line 7 <div>{data.site.siteMetadata.pageHeading}</div>
the React component is taking data out of the queried properties, in our case specifically the siteMetadata.pageHeading
property and inserting it into the HTML. This is one of the benefits of JSX, write any JS expression in the view the only requirement is wrapping the JS in {}
. Pretty exciting stuff here. Now we can update the other 2 pages as follows:
page1.js
import * as React from "react";
import { graphql } from "gatsby";
const PageOne = ({ data }) => {
return (
<main>
<div>{data.site.siteMetadata.pageHeading}</div>
<div>Page 1</div>
</main>
);
};
export const query = graphql`
query indexPageQuery {
site {
siteMetadata {
pageHeading
}
}
}
`;
export default PageOne;
page2.js
import * as React from "react";
import { graphql } from "gatsby";
const PageTwo = ({ data }) => {
return (
<main>
<div>{data.site.siteMetadata.pageHeading}</div>
<div>Page 2</div>
</main>
);
};
export const query = graphql`
query indexPageQuery {
site {
siteMetadata {
pageHeading
}
}
}
`;
export default PageTwo;